Manipulation of raw binary payloads in WarpScript could be done easily with existing functions, no need to call external programs!
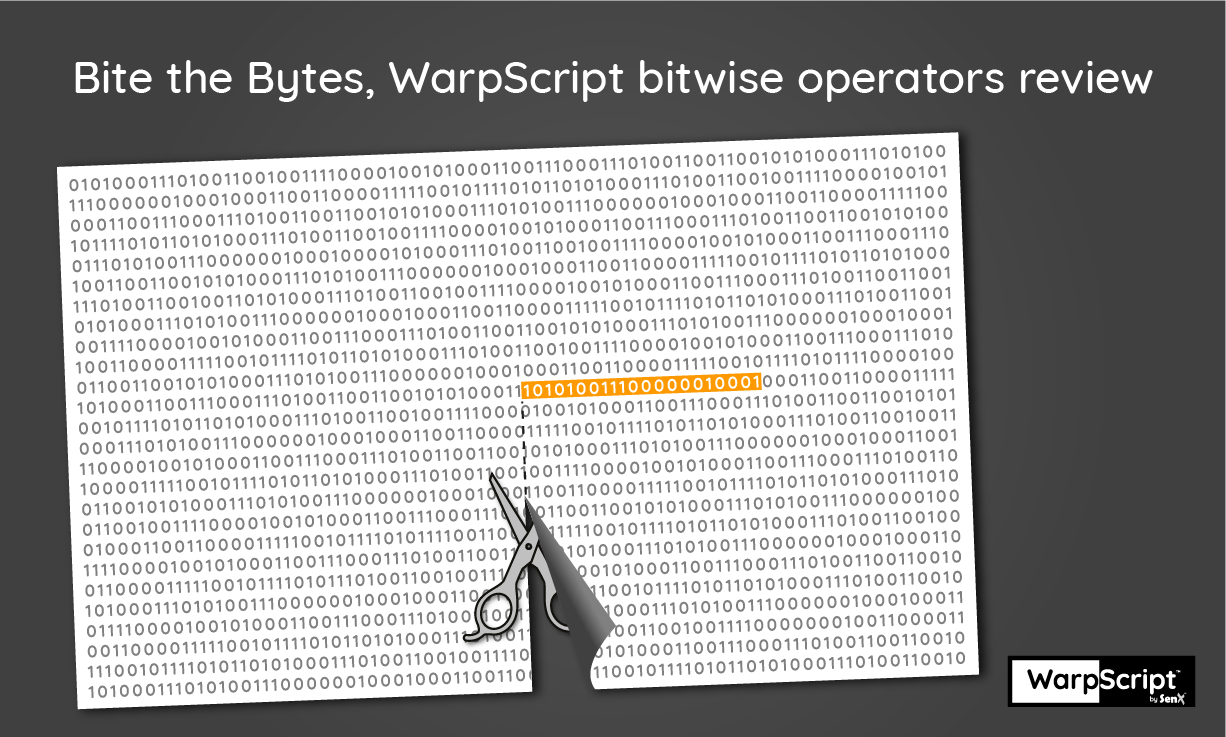
Sometimes, you need to process raw payloads. Extract a bit from an array of bytes. Build a byte array. If you play with Warp 10 MQTT plugin (now available on WarpFleet), you have to manipulate the payload you build on your IoT device.
Here is a useful cheat-sheet for bit and bytes manipulation in WarpScript.
Use LONG, BYTES
Most conversions from and to LONG or BYTES (bytes array) type exists. We will take an 8 bytes CAN frame as example payload. It contains two booleans, an integer, and a float:
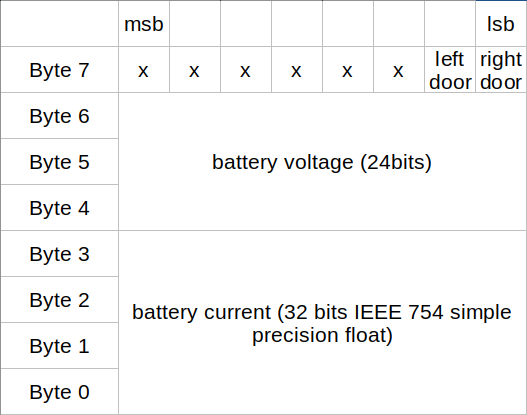
Analyze your electrical consumption from your Linky and Warp 10 |
This could be described in a C struct, using bit fields:
struct {
unsigned int right_door_opened: 1;
unsigned int left_door_opened: 1;
char: 0; //padding
int batteryVoltage: 24;
float batteryCurrent;
};
Decode payloads
payload.right_door_opened = 1;
payload.left_door_opened = 0;
payload.batteryVoltage = 351;
payload.batteryCurrent = -140.424;
The encoded frame could be represented as a long with value -4392016192336732415
, or c30c6c8b00015f01
in hexadecimal. In WarpScript, you could decode everything in a few lines:
Extract a byte array from a byte array | SUBSTRING |
Read a byte from a byte array, returns a long | GET |
Convert a byte array to a long | TOLONG |
Convert a byte array to a float | TOLONG FLOATBITS-> |
Convert a byte array to a double | TOLONG DOUBLEBITS-> |
Convert a byte array to a string | "UTF-8" BYTES-> |
Read a bit from a byte array, returns a boolean | BYTESTOBITS 56 BITGET |
Reverse a byte array endianness | REVERSE |
Of course, if you define the same C struct on another CPU, you might have to reverse endianness somewhere!
Encode payloads
Here is the code to build the byte array with the same content as the previous example:
Again, convert what you need to long, then to byte arrays, and use +
to concatenate byte arrays. For booleans, use bitwise shift <<
and binary mask |
and &
to build long.
Convert a long to a byte array, from 1 to 8 bytes | 3 ->LONGBYTES |
Concatenate byte arrays together | + |
Convert a float to a byte array | ->FLOATBITS 4 ->LONGBYTES |
Convert a double to a byte array | ->DOUBLEBITS 8 ->LONGBYTES |
Reverse a byte array endianness | REVERSE |
Debug
WarpScript also includes functions to represent byte array as strings. These functions are useful for debugging, checking endianness problems, and so on.
Display byte array in hexadecimal | ->HEX |
Display byte array in binary | ->BIN |
Display byte array in base64 | ->B64 |
Decode a byte array | 'UTF-8' BYTES-> |
Conclusion
Like many languages, WarpScript allows to build or decode any kind of binary payload. If you want to check all available type conversions, read this tutorial!
Read more
All about the Geo Time Series Model
Saving and processing sensor data with Node-RED and WarpScript
Working with GEOSHAPEs: code contest!
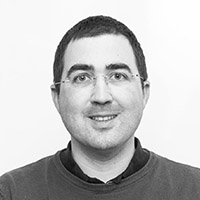
Electronics engineer, fond of computer science, embedded solution developer.