Ever wonder how to perform HTTP requests from WarpScript? Depending on your needs, here are 3 ways to achieve it.
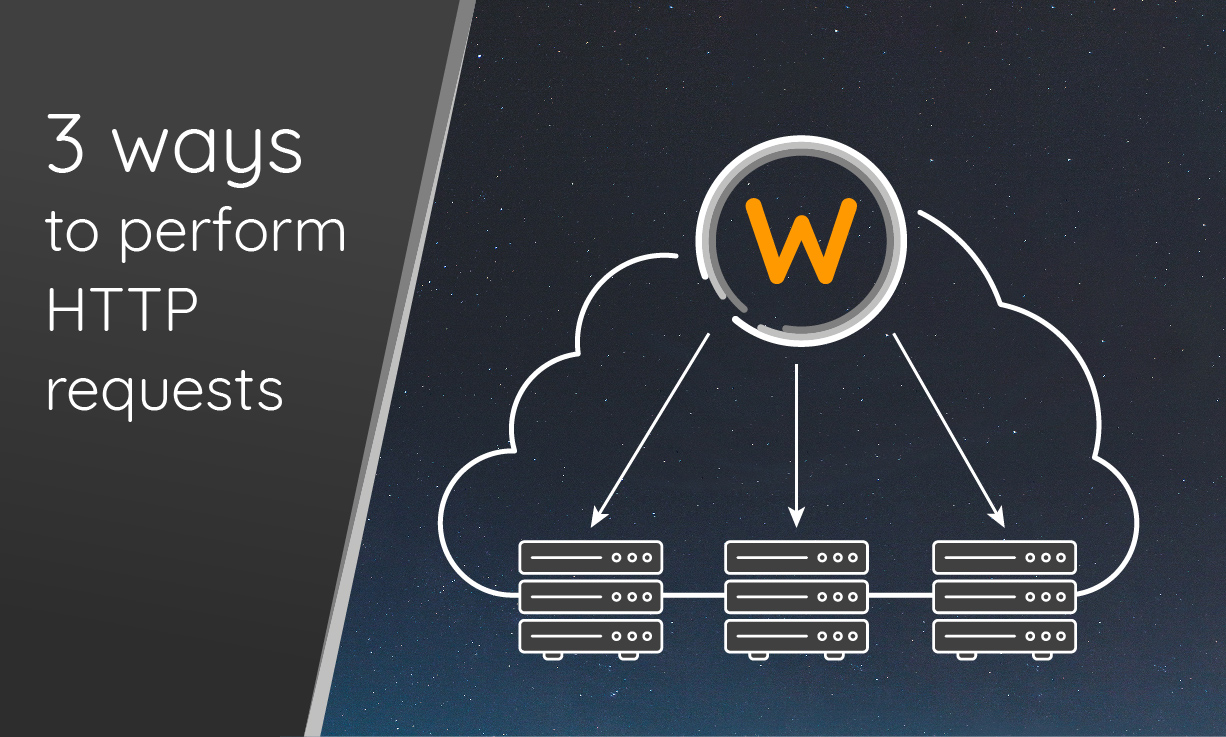
Often we need to perform HTTP requests in our scripts, for instance, to send a notification in a Slack channel or to a mailing API, to fetch data from a REST API or to send data to a tier server.
There are 3 ways to perform HTTP requests in WarpScript or FLoWS.
WEBCALL
WEBCALL is historically the first one. It allows you to send an HTTP request without waiting for a response. This is a one-way action. WEBCALL is handy to notify an HTTP Server without slowing your code execution or introducing a bottleneck.
Configuration parameters in /path/to/warp10/etc/conf.d/10-webcall.conf
:
webcall.host.patterns
: allow/disallow some hosts based on regular expression likeslack.com,!^127.0.0.1$,!^localhost$,^192.168.*
warpscript.maxwebcalls
: maximum parallel usagehttp.header.webcall.uuid
: will appear in theX-Warp10-WebCall
headerwebcall.user.agent
: "Warp10-WebCall" by default
WarpScript
'9M8DP97xxxxxxZwKm78zc' // WRITE token
'POST'
'https://hooks.slack.com/services/T01xxxxNT1/B0xxxM/bzZxxx5yHdJJ'
{ 'Content-Type' 'application/json' } // HTTP headers
{
'channel' '#alertes'
'username' 'Warp 10'
'text' "Published in #alertes from user Warp 10."
'icon_emoji' ':spock-hand:'
} ->JSON // Payload
WEBCALL
FLoWS
payload = ->JSON({
"channel": "#alertes",
"username": "Warp 10",
"text": "Published in #alertes from user Warp 10.",
"icon_emoji": ":spock-hand:"
})
return WEBCALL(
"9M8DP97xxxxxxZwKm78zc", // WRITE token
"POST",
"https://hooks.slack.com/services/T01xxxxNT1/B0xxxM/bzZxxx5yHdJJ",
{ "Content-Type": "application/json" },
payload
)
URLFETCH
URLFETCH allows you to perform a GET request and give you back the response, headers and status. It could be a bottleneck in your code, because it waits for the response.
For security reasons, you must be authenticated with a READ token.
By the way, this function will be deprecated in a future Warp 10 version because replaced by HTTP
.
Configuration parameters in /path/to/warp10/etc/conf.d/70--extensions.conf
:
warpscript.extension.urlfetch = io.warp10.script.ext.urlfetch.UrlFetchWarpScriptExtension
: Activate the extensionwarpscript.urlfetch.limit
: maximum parallel usage a developer can bypasswarpscript.urlfetch.limit.hard
: maximum parallel usagewarpscript.urlfetch.maxsize
: maximum response size a developer can bypasswarpscript.urlfetch.maxsize.hard
: maximum response sizewarpscript.urlfetch.host.patterns
: allow/disallow some hosts based on regular expression likeslack.com,.*!
WarpScript
'oQ4t3FJSguxxxxxxxxxxUCXo3vvRGg0Q5.' // READ token
AUTHENTICATE
'https://random-data-api.com/api/name/random_name?size=5' URLFETCH 0 GET 3 GET // get body
B64-> 'utf8' BYTES-> JSON-> // decode as JSON
<% 'name' GET %> F LMAP
FLoWS
AUTHENTICATE('oQ4t3FJSguxxxxxxxxxxUCXo3vvRGg0Q5.')
response = URLFETCH('https://random-data-api.com/api/name/random_name?size=5')
body = JSON->(BYTES->(B64->(response[0][3]), 'utf8')) // extract body as JSON
return LMAP(body, (item)-> { return item['name'] }, false)
HTTP
HTTP allows you to send an HTTP request (thanks Captain Obvious), waiting for a response but with more options than URLFETCH, especially, you can specify the method.
One more important think, you can even stream your data using the chunks.
Configuration parameters in /path/to/warp10/etc/conf.d/70--extensions.conf
:
warpscript.extension.http=io.warp10.script.ext.http.HttpWarpScriptExtension
: Activate the extensionwarpscript.http.authentication.required
: allows you to bypass the authentification process (which is mandatory for URLFETCH)warpscript.http.capability
: Token capabilities handlingwarpscript.http.maxrequests
: maximum parallel usagewarpscript.http.maxsize
: maximum response sizewarpscript.http.maxchunksize
: maximum chunk sizewarpscript.http.host.patterns
: allow/disallow some hosts based on regular expression likeslack.com,.*!
WarpScript
{
'url' 'https://hooks.slack.com/services/T01xxxxNT1/B0xxxM/bzZxxx5yHdJJ'
'method' 'POST'
'headers' { 'Content-Type' 'application/json' }
'body' {
'channel' '#alertes'
'username' 'Warp 10'
'text' "Published in #alertes from user Warp 10."
'icon_emoji' ':spock-hand:'
} ->JSON
}
HTTP
FLoWS
return HTTP({
"url": "https://hooks.slack.com/services/T01xxxxNT1/B0xxxM/bzZxxx5yHdJJ",
"method": "POST",
"headers": { "Content-Type": "application/json" },
"body": '{
"channel": "#alertes",
"username": "Warp 10",
"text": "Published in #alertes from user Warp 10.",
"icon_emoji" ":spock-hand:"
}'
})
Going further
You can now access external APIs and make Warp 10 dialog with your stack.
To simplify your usage, you can develop macros to embed those calls in order to factorize and control your code.
Read more
Health data analysis made easy with Warp 10
Captain's Log, Stardate 4353.3
Using Warp 10 as a map tile server for Discovery
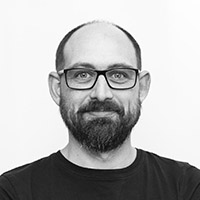
Senior Software Engineer